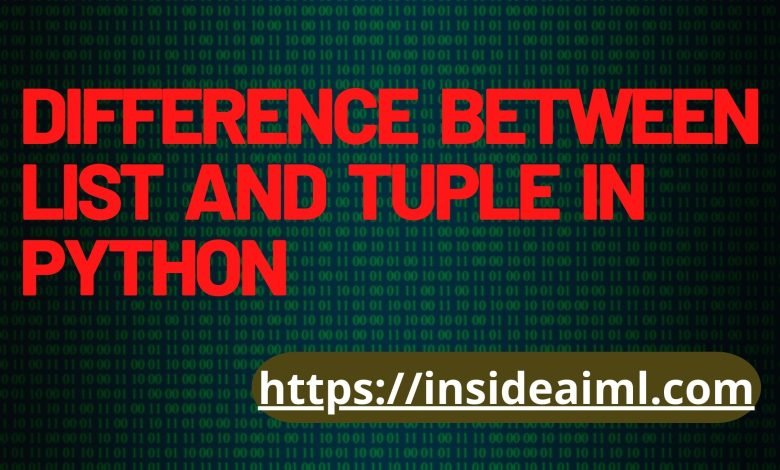
Difference between list and tuple in python
One or more objects or values can be stored in a list or tuple, and that order is preserved. Any type, even the Nothing type defined by the None Keyword, can be used for the objects that make up a list or tuple.
In this post, we’ll explore the tuple vs list, two data structures that are otherwise very similar.
Lists
Lists are one of the primary data structures in Python, and their purpose is to store collections of related objects. List to tuple Python, like arrays, helps group values of the same type together, reducing the amount of code needed to manipulate them. If you save your music in a folder on your desktop, for instance, you can organize it into subfolders based on genre. Python’s list-to-tuple conversion is used to better manage all the values in the system and increase efficiency.
Tuples:
Tuples-like lists can be used to store several things sequentially. Separating each item is a comma. Once a tuple is generated, it cannot be changed and no new items can be added to it. Tuples, which limit the collection, also prevent the removal of pieces. The advantage of immutability is shown most clearly in the form of quicker, more effective results.
Tuple and list Python share the same fundamental purpose and structure, but their implementations are distinct. What you’ll learn in this blog post about tuples and lists in Python:
what is the difference between list and tuple in python?
Lists are mutable sequences that have several methods (both mutating and non-mutating) and are typically used as generic containers (whose contents can be objects of any type, while it is sometimes regarded better style for lists to have contents of the same type or kinds to be used equivalently).
To use a container as a member of a set or a key in a dict, you’ll want to work with a tuple, which is an immutable sequence with relatively few methods (all non-mutating special ones) (though the items will also have to be immutables. Objects of any type can be included in tuples; in fact, it’s rather common for a single tuple to contain items of a wide variety of types.
In the rare instances where a list and a tuple are functionally equivalent, the former can be preferred due to its smaller size and faster construction time. In cases where the function must return numerous values, for instance,
list and tuple difference in python.
Python’s List and Tuple Structures: What You Need to Know
List and tuple are both Python data structures that can be used to store and retrieve information, but they differ significantly. In this case, the list is highly variable, while the elements of a tuple are fixed. Tell us more information about both of these.
Important details to keep in mind:
- Parentheses () indicate the literal syntax of tuples, while square brackets [] indicate the literal syntax of lists.
- Tuples have a set length but lists do not.
- In contrast to immutable tuples, lists can be changed.
- The list outperforms the tuple in terms of features.
Comparison of Python Lists vs Tuples for Similarities
Now that we’ve revisited tuples and lists, we can start by talking about what they have in common. Listed here are some parallels:
similarity between a list and a tuple is that they are both sequence types that may store multiple values.
- Both of them are capable of storing information in various formats.
- The index can be used to look up both the list and tuple elements.
- we can layer both tuples and lists, creating nested tuples and lists.
For the time being, let’s focus on what sets Python Lists and Tuples apart from one another.
1 Dissimilarity in Syntax
When a list is written, its elements are enclosed in square brackets, while tuples use round brackets.
2. Size
The Python programming language’s tuples take up less space than lists. Due to the immutability of dice tuples, more space can be reserved for them with less overhead. However, lists are given much smaller chunks of memory. This trait also makes tuples faster than the lists, when they have huge numbers of elements.
3. Mutability
This is one of the main differences between the lists and the tuples. In Python, lists are mutable although tuples are not.
4. Functions and Methods
Several functions and methods are common to both the lists and the tuples. These are len(), max(), min(), sorted(), sum() all(), any(), index(), and count() ().
These are the functions that do not affect the constructions. Many more built-in methods are not widespread. These are the functions that change the structures like add(), del, etc.
5. Tuples in Lists and Lists in Tuples
We can utilize tuples as elements of the lists and vice versa. This is done to boost the readability of the values.
6. Length
The length of a tuple is fixed whereas the length of a list is flexible. The explanation again is the immutability feature.
7. Debugging
Tuples are often used for major projects when we know the information need not be modified. This is because tuples are mutable and so it is easier to track them. This makes them programmer-friendly when it comes to debugging as compared to the lists. For simpler tasks, you can utilize lists.
8. Usage
Lists are used to keep a list of homogeneous elements. And tuples are utilized if we store heterogeneous items like details of a person/user. There is no restriction on this, but this is merely a general tendency followed.
Lists are useful in circumstances where we need to alter or delete the values. Whereas the tuples are employed in circumstances where we supply read-only property.
Dictionary keys are another popular example of a context in which tuples are useful but lists are not. Always keep in mind that a dictionary is a data structure that stores information in the form of key-value pairs. It’s safe to assume that the keys of a dictionary won’t ever change. Because the keys are immutable, tuples can be used effectively.
What is the difference between list and tuple in python, and an array as a data structure?
Talking about “arrays” and “lists” as abstract data types without reference to any particular implementation can be a bit confusing because those two are not very clearly defined.
The two Wikipedia pages List (abstract data type) and Array data type make this ambiguity relatively clear, with questionable phrases such as “Implementation of the list data structure may include some of the following operations:”.
In many languages, there is a type called list and a type named array and they have some well-defined meanings.
Here is a very general summary.
Lists:
- A list is linear, it is an ordered series of elements;
- You can access the first element of a list; You can add a new element to a list;
- You can access all elements of the list one after the other, starting from the first element.
- That last step is done either with “arbitrary access” (accessing elements as l[0], l[1], l[2], etc.) or with two operations termed “head” and “tail”, where the head(l) returns the first element of l and tail(l) returns the sublist generated by discarding the first element.
Conclusion
In this essay, we have described the difference between list and tuple in python. Then we saw similarities and differences in tuples and lists in Python. We also witnessed the uses of each of them in different scenarios.
Hoping that you grasped the sub-topics addressed. Happy learning.