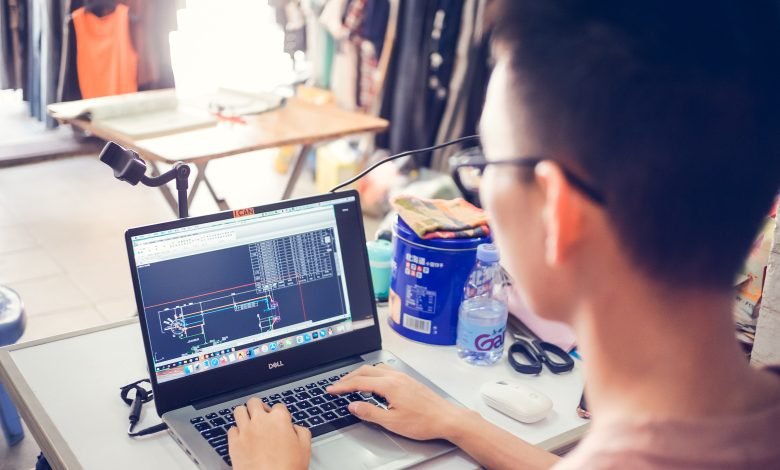
Data Structure in Python
Data structure basically means organizing, managing and storing data in Python which is very important to understand if you are pursing Python course in Delhi. So let’s know about data structure in detail in this blog.
With the help of data structure, we organize our data in ways like storing data, relate them and perform operation.
If we talk about the types of data structure then yes they exist in Python.
So the first type is Non-Primitive data structures which mainly store the data in the form of collection of values in various formats. The list of common one is given below.
- List
- Tuple
- Dictionaries
- Sets
Let’s read about these non-primitive data structures in detail.
List: List is used to store multiple items in a single variable. List items are ordered, changeable and have duplicate values.
For e.g.
Mylist =[“apple”, ”banana”, ”orange”]
Print (Mylist)
[‘apple’, ’banana’, ’orange’]
Lets’s discuss some Important points about LIST:
1. In list to find the length of items Len() function is used.
2. A list contains different data types:
mylist = [“abc”,10,True,20,”male”]
Print(mylist)
[‘abc’,10,True,20,’male’)
3. To find the type of list <class ‘list’> is used.
Tuple: Tuple is a collection which is ordered, unchangeable, and allows duplicate members.
For e.g.
mytuple = (“red”, ”blue”, ”green”)
print(mytuple)
(‘red’, ’blue’, ‘ green’)
To print the length of the tuple –
Print(len(mytuple))
3
Tuple items can be of any data types.
Set: Set doesn’t support duplicate members in the collection and it makes an unordered and unchangeable collection.
Creating a Set
myset = {“apple”, “banana”, “orange”)
Print(myset)
{‘apple’, ’banana’, ’orange’}
Set items can be of any type:
For e.g.
myset = {“apple”, 20 ,True}
print(myset)
{‘apple’,20,True}
Dictionary: Dictionary is a collection which is ordered and changeable and doesn’t allow duplicate members. This type is used to store data values in key: value pairs. Always remember, dictionaries don’t have same key for different items.
For e.g.
thisdict = { “fruit” : “apple”,
“color” : “red”,
}
Print(thisdict[“color”]
Red
Print(len(thisdict))
2
Print(type(thisdict))
<class ‘dict’>
Python has another type of data structures known as Primitive data structures. Their list is given below.
- Integer
- Boolean
- Float
- String
Interger – This data type is used to present numerical data that is either positive or negative.
Like -1, 3, or 6.
Boolean – The Boolean expressions take the values True or False.
Like –
The true values are
1==1 is True
The false values are
2<1 is false
Float – Float represent as floating-point real numbers, usually contains a decimal point.
Like– 2.0 or 5.77.
String – This data types use collection of words and alphabets etc. Single or double quotes are used. Two concatenate two or more values “+” operators is used.
Like-‘hello’,’welcome’etc.
Let’s talk about the very popular term in Python and web programming that is Loops.
LOOPS
FOR LOOPS
It is used for restating over a sequence.
(That can be a list, a tuple, a dictionary, or a set)
For e.g.
Colors = [“red”, “blue”, “pink”]
For x in colors :
Print(x)
Red
Blue
Pink
RANGE FUNCTION IN FOR LOOP
The range () function returns a sequence of numbers, starting from 0 by default.
For e.g.
for x in range(5):
Print(x)
0
1
2
3
4
ELSE IN FOR LOOP
It prints the all members mentioned in the program and also prints the message that when the loop has ended.
For e.g.
for x in range (5):
print(x)
else :
print (“finally finished”)
0
1
2
3
4
5
Finally finished!
WHILE LOOP
The while loop will execute a set of statements as long as a condition is true.
For e.g.
I = 1
while I < 6 :
Print (i)
I+=1
1
2
3
4
5
The above example shows that it print “I” as long as “I” is less than 6.
so let’s talk in detail then we find that while loop first checks the condition and then executes the statements. For example, if the expression is true, the statement inside the body will execute. This process will continue until it evaluated false. If expression is false the loop terminates end.
With Python, you work with DJANGO and forms are very important to understand in it. Let’s know about it in detail.
Working with Django to create forms
So let’s Follow the given below steps.
- Create a forms.py file in your application
- Afetr that import forms from django
- After that Create a view for the form
- so let’s finally from django.forms import form
def form(request)
return render(request,’Form.html’, {“form”: form})
- In the templates a HTML file will be created for displaying the form.
- Connect the view in urls.py file.
The process for creating a url for the view is-
- From django.conf.urls import views library is used.
- Afetr this we have to declare a url values in urlpattern list
Urlpatterns = [here url_path , view , name of view to be mentioned ]
From django.contrib import admin.
After that import url from django.conf.urls
Final Step import views From django
urlpatterns=[
path(‘formpage/’,views.form,name=’form’),
url(‘admin-panel/’, admin.site.urls), ]
- Let’s Finally run the server by python manage.py runserver
There are some form fields which is used for creating django form.
For e.g.
- forms.BooleanField() – which creates the Boolean field.
- forms.CharField() – It creates a text field.
- forms.EmailField() – It creates an text for email.
- forms.URLField() – It allows the url
- forms.ImageField() – In this a field to be created in which user can attach their images.
Similarly, this was a quick discussion on the Python data structures, loops, and creating forms using Django. For working on Python in more detail you can go for Python data analytics course in both online or classroom modes of learning.
Read more blogs on – https://articlesdo.com/